Print & Format
Formatting output data with Print & Format
Print & Format
In a previous tutorial (Serial Port and RS-232 for communication) You probably noticed it is annoying to have to change RealTerm from ASCII to HEX in order to see output of numbers. It is also not easy to view raw HEX data. You will probably want to be able to output decimal numbers to make it easy on us humans. This is where the print and format libraries come in!
The print and format libraries are quite easy to use, start off by including them into your main file (after your serial port include block).
include print include format
Of course, now you need to know what procedures are available. This is when it is a good idea to open up a library file. Scroll through the file and note procedure names as well as their input parameters.
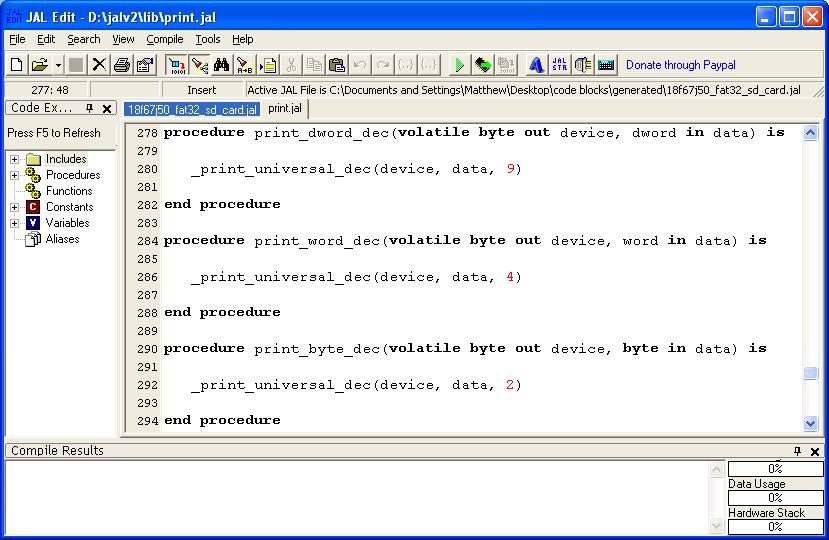
Print and format numbers
In the previous image, you can see 3 procedure names:
- print_byte_dec() - Prints a byte in decimal format.
- print_word_dec() - Prints a word in decimal format.
- print_dword_dec() - Prints a dword in decimal format.
Each requires the following parameters:
- device - The device the data will be outputted to. Usually you will put serial_hw_data or serial_sw_data. However, you can put any pseudo variable (fake variable, that is actually a procedure) as an input. This pseudo variable usually allows writing to an output device such as and LCD or protocol SPI, I2C, etc.
- data - The data to be sent to the device.
The following example will set the value of the word x to 543 and send it to the serial serial port in ASCII format:
var word x = 543 print_word_dec(serial_hw_data, x)
Now let's have a look at the format library. you will see 3 alike procedures with names:
- format_byte_dec - Formats a byte in decimal.
- format_word_dec - Formats a word in decimal.
- format_dword_dec - Formats a dword in decimal.
These format procedures are able to format a byte, word or dword. Here are the input parameters:
- device - Same as print library.
- data - The data to be sent to the device.
- n_tot - The total length of the outputted number (Including sign' +/-', and decimal point)
- n2 - The number of decimal places.
The following example will write 61.234 to the serial port in ASCII format:
var dword_dec x = 61234 format_dword_dec(serial_hw_data,x,6,3)
Printing Strings
The print library also has a procedure for printing strings. called print_string. It requires 2 inputs:
- device - same as above.
- str[] - The string to print to the serial port (an array of characters).
Here's an example that will output "Hello World" to the serial port in ASCII format:
const byte hello_string[] = "Hello World" print_string(serial_hw_data, hello_string)
Last of course, you may need to go to the next line with carriage return + line feed (CRLF).
print_crlf(serial_hw_data)
CRLF can also be put directly into your string with "\r\n". This will put Hello and World on 2 separate lines.
const byte hello_string[] = "Hello\r\nWorld" print_string(serial_hw_data, hello_string)
Put it all together
include 16f877a -- target PICmicro -- -- This program assumes a 20 MHz resonator or crystal -- is connected to pins OSC1 and OSC2. pragma target clock 20_000_000 -- oscillator frequency -- configure fuses pragma target OSC HS -- HS crystal or resonator pragma target WDT disabled -- no watchdog pragma target LVP disabled -- no Low Voltage Programming enable_digital_io() -- disable analog I/O (if any) -- ok, now setup serial const serial_hw_baudrate = 115_200 include serial_hardware serial_hw_init() include print include format const byte start[] = "Start of main program...\r\n" print_string(serial_hw_data,start) var dword x = 61234 const byte string1[] = "Let's print a dword: " print_string(serial_hw_data,string1) print_dword_dec(serial_hw_data,x) print_crlf(serial_hw_data) const byte string2[] = "Let's print a dword with 3 decimal places: " print_string(serial_hw_data,string2) format_dword_dec(serial_hw_data,x,6,3) print_crlf(serial_hw_data) const byte string3[] = "Let's print it in hex: " print_string(serial_hw_data,string3) print_dword_hex(serial_hw_data,x) print_crlf(serial_hw_data) const byte string4[] = "If we print as a hex byte, it will be truncated: " print_string(serial_hw_data,string4) print_byte_hex(serial_hw_data, byte(x) ) const byte end[] = "\r\nEnd of main program..." print_string(serial_hw_data,end)
Here's the output. Take special note of how and why our number 61234 in hex (0x0000EF32) got reduced into a byte (0x32) in the 5th line shown below.
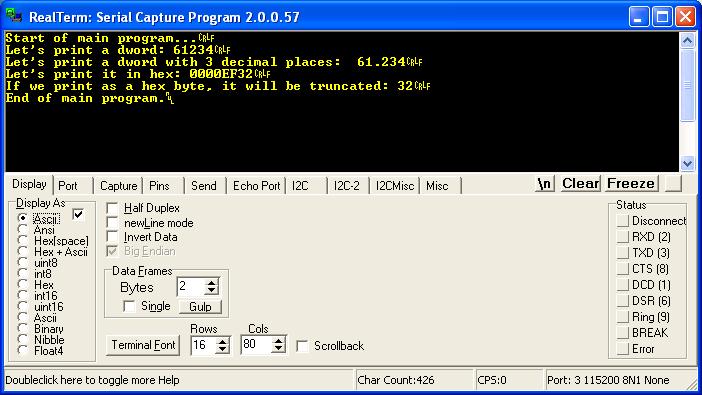
There you go... that's print and format! Doesn't this make life so easy!